Elvis abuse, also known as the Elvis operator or null coalescing operator, is a syntactic construct in many programming languages that provides a concise way to handle null values.
The Elvis operator is typically represented by the ?:
symbol. Its syntax is as follows:
variable = expression1 ?: expression2
If expression1
is not null, the value of variable
will be set to expression1
. Otherwise, the value of variable
will be set to expression2
.
The Elvis operator is a powerful tool that can be used to simplify code and improve readability. It can be used in a variety of situations, such as:
- Assigning a default value to a variable
- Checking for null values before performing an operation
- Chaining multiple Elvis operators together to handle complex null cases
The Elvis operator is a valuable addition to any programming language. It can help to write more concise, readable, and maintainable code.
Elvis Abuse
Elvis abuse, also known as the Elvis operator or null coalescing operator, is a syntactic construct in many programming languages that provides a concise way to handle null values.
- Simplicity: The Elvis operator is a simple and concise way to handle null values.
- Readability: The Elvis operator makes code more readable and easier to understand.
- Maintainability: The Elvis operator helps to write more maintainable code by reducing the number of null checks.
- Performance: The Elvis operator can improve the performance of code by avoiding unnecessary null checks.
- Versatility: The Elvis operator can be used in a variety of situations, such as assigning default values to variables, checking for null values before performing an operation, and chaining multiple Elvis operators together to handle complex null cases.
- Language Agnostic: The Elvis operator is available in many programming languages, making it a valuable tool for developers of all levels.
- Popularity: The Elvis operator is a popular feature in many programming languages, indicating its usefulness and widespread adoption.
- Cross-Platform: The Elvis operator is supported in many programming languages that run on a variety of platforms, making it a portable and versatile tool.
- Community Support: The Elvis operator has a strong community of support, with many resources and tutorials available online.
Overall, the Elvis operator is a valuable tool that can help developers write more concise, readable, maintainable, and performant code. It is a versatile and popular feature that is supported in many programming languages.
Simplicity
The simplicity of the Elvis operator is one of its key benefits. It provides a concise and easy-to-understand way to handle null values. This can greatly improve the readability and maintainability of code.
For example, consider the following code:
String name = person.getName();if (name == null) { name ="John Doe";}
This code uses a traditional if statement to check for a null value and assign a default value if necessary. However, this code can be simplified using the Elvis operator:
String name = person.getName() ?: "John Doe";
This code is much more concise and easier to read. It also eliminates the need for the if statement, which can improve the performance of the code.
The simplicity of the Elvis operator makes it a valuable tool for developers. It can help to write more concise, readable, and maintainable code.
Readability
The readability of code is an important factor in software development. Code that is easy to read is easier to understand, maintain, and debug. The Elvis operator can help to improve the readability of code by providing a concise and easy-to-understand way to handle null values.
For example, consider the following code:
String name = person.getName();if (name == null) { name ="John Doe";}
This code uses a traditional if statement to check for a null value and assign a default value if necessary. However, this code can be simplified using the Elvis operator:
String name = person.getName() ?: "John Doe";
This code is much more concise and easier to read. It also eliminates the need for the if statement, which can improve the performance of the code.
The Elvis operator can be used in a variety of situations to improve the readability of code. For example, it can be used to:
- Assign default values to variables
- Check for null values before performing an operation
- Chain multiple Elvis operators together to handle complex null cases
The Elvis operator is a valuable tool for developers who want to write more readable and maintainable code.
In conclusion, the readability of code is an important factor in software development. The Elvis operator can help to improve the readability of code by providing a concise and easy-to-understand way to handle null values.
Maintainability
Maintainability is an important aspect of software development. Maintainable code is easy to read, understand, and modify. The Elvis operator can help to improve the maintainability of code by reducing the number of null checks.
- Reduced Code Complexity
The Elvis operator can reduce the complexity of code by eliminating the need for explicit null checks. This can make code easier to read and understand, and it can also reduce the number of bugs.
- Improved Error Handling
The Elvis operator can help to improve error handling by providing a concise and consistent way to handle null values. This can make it easier to identify and fix errors, and it can also help to prevent errors from propagating through the code.
- Increased Code Reusability
The Elvis operator can help to increase the reusability of code by providing a way to handle null values that is independent of the specific programming language or framework being used. This can make it easier to reuse code across different projects and applications.
Overall, the Elvis operator is a valuable tool for developers who want to write more maintainable code. It can help to reduce the complexity of code, improve error handling, and increase code reusability.
Performance
The Elvis operator can improve the performance of code by avoiding unnecessary null checks. This is because the Elvis operator short-circuits the evaluation of the second expression if the first expression is not null. This can result in significant performance improvements, especially in code that frequently checks for null values.
- Reduced Number of Instructions
The Elvis operator can reduce the number of instructions that are executed by avoiding unnecessary null checks. This can result in significant performance improvements, especially in code that frequently checks for null values.
- Improved Cache Locality
The Elvis operator can improve cache locality by reducing the number of memory accesses that are required to check for null values. This can result in significant performance improvements, especially in code that frequently checks for null values in large data structures.
- Increased Concurrency
The Elvis operator can increase concurrency by reducing the number of locks that are required to check for null values. This can result in significant performance improvements, especially in code that frequently checks for null values in multithreaded applications.
Overall, the Elvis operator is a valuable tool for developers who want to write high-performance code. It can help to reduce the number of instructions that are executed, improve cache locality, and increase concurrency.
Versatility
The versatility of the Elvis operator is one of its key strengths. It can be used in a variety of situations to handle null values, making it a valuable tool for developers.
One common use of the Elvis operator is to assign default values to variables. For example, the following code assigns the default value "John Doe" to the variable name
if it is null:
String name = person.getName() ?: "John Doe";
The Elvis operator can also be used to check for null values before performing an operation. For example, the following code checks if the variable name
is null before printing it to the console:
if (name != null) { System.out.println(name);}
The Elvis operator can also be chained together to handle complex null cases. For example, the following code assigns the default value "John Doe" to the variable name
if it is null, and then checks if the variable age
is null before printing it to the console:
String name = person.getName() ?: "John Doe";if (age != null) { System.out.println(age);}
The versatility of the Elvis operator makes it a valuable tool for developers. It can be used to simplify code, improve readability, and handle null values in a variety of situations.
The versatility of the Elvis operator is also important because it allows developers to write code that is more robust and less error-prone. By using the Elvis operator to handle null values, developers can avoid the NullPointerException, which is a common error in Java programming.
Overall, the versatility of the Elvis operator is one of its key strengths. It makes the Elvis operator a valuable tool for developers who want to write code that is concise, readable, and robust.
Language Agnostic
The Elvis operator is a valuable tool for developers of all levels because it is available in many programming languages. This means that developers can use the Elvis operator to write code that is portable and reusable across different platforms and applications. For example, the Elvis operator is available in Java, Kotlin, Groovy, Scala, and many other programming languages.
The Elvis operator can be used to improve the readability and maintainability of code. By using the Elvis operator to handle null values, developers can avoid the NullPointerException, which is a common error in programming. The Elvis operator can also be used to simplify code and to make it more concise.
Overall, the Elvis operator is a valuable tool for developers of all levels. It is a versatile and portable operator that can be used to improve the readability, maintainability, and performance of code.
Popularity
The popularity of the Elvis operator is a testament to its usefulness and widespread adoption. Developers have recognized the benefits of the Elvis operator, such as its simplicity, readability, maintainability, performance, and versatility. As a result, the Elvis operator has become a popular feature in many programming languages.
The popularity of the Elvis operator has also led to its widespread adoption. Developers are increasingly using the Elvis operator to handle null values in their code. This is because the Elvis operator provides a concise and easy-to-understand way to handle null values. As a result, the Elvis operator has become a valuable tool for developers of all levels.
The popularity and widespread adoption of the Elvis operator has had a significant impact on the way that developers write code. The Elvis operator has helped to improve the readability, maintainability, and performance of code. As a result, the Elvis operator has become an essential tool for developers who want to write high-quality code.
Cross-Platform
The cross-platform nature of the Elvis operator is a key factor in its widespread adoption and popularity. Developers can use the Elvis operator to write code that is portable and reusable across different platforms and applications. This is a significant advantage, especially in today's world where applications are increasingly being deployed on multiple platforms.
- Portability
The Elvis operator is portable across different platforms because it is implemented in many programming languages. This means that developers can write code that uses the Elvis operator and be confident that it will run on any platform that supports the programming language.
- Reusability
The Elvis operator is reusable across different applications because it provides a consistent way to handle null values. This means that developers can write code that uses the Elvis operator and be confident that it will work correctly in any application.
- Improved Code Quality
The Elvis operator can help to improve the quality of code by making it more readable and maintainable. This is because the Elvis operator provides a concise and easy-to-understand way to handle null values.
- Increased Productivity
The Elvis operator can help to increase developer productivity by reducing the amount of time that is spent writing and debugging code. This is because the Elvis operator provides a simple and effective way to handle null values.
Overall, the cross-platform nature of the Elvis operator is a significant advantage that makes it a valuable tool for developers. The Elvis operator can help to improve the portability, reusability, quality, and productivity of code.
Community Support
The strong community support for the Elvis operator is a major factor in its widespread adoption and popularity. Developers can find a wealth of resources and tutorials online, making it easy to learn how to use the Elvis operator effectively. This community support also ensures that the Elvis operator is well-maintained and up-to-date.
The availability of community support is essential for the success of any programming language feature. Developers need to be able to find resources and tutorials to learn how to use the feature, and they need to be able to get help when they encounter problems. The strong community support for the Elvis operator makes it easy for developers to learn how to use the feature and to get help when they need it.
The practical significance of this understanding is that developers can be confident that the Elvis operator is a well-supported feature that they can use to improve the quality of their code. Developers can find a wealth of resources and tutorials online to learn how to use the Elvis operator effectively, and they can get help from the community when they encounter problems.
Frequently Asked Questions about Elvis Abuse
Elvis abuse is a serious issue that can have a devastating impact on the lives of those who experience it. It is important to be aware of the signs and symptoms of Elvis abuse, and to know how to get help if you or someone you know is being abused.
Question 1: What is Elvis abuse?
Elvis abuse is a form of domestic violence that involves the use of physical, emotional, or sexual violence by one intimate partner against another. It can also involve controlling or coercive behavior, such as isolation, threats, or financial abuse.
Question 2: What are the signs and symptoms of Elvis abuse?
The signs and symptoms of Elvis abuse can vary depending on the type of abuse, but some common signs include:
- Physical injuries, such as bruises, cuts, or broken bones
- Emotional distress, such as anxiety, depression, or PTSD
- Sexual abuse, such as forced sex or unwanted sexual contact
- Controlling or coercive behavior, such as isolation, threats, or financial abuse
Question 3: What are the causes of Elvis abuse?
The causes of Elvis abuse are complex, but some risk factors include:
- A history of violence in the family
- Substance abuse
- Mental health issues
- Poverty
- Lack of social support
Question 4: What are the consequences of Elvis abuse?
Elvis abuse can have a devastating impact on the lives of those who experience it. It can lead to physical injuries, emotional distress, sexual health problems, and even death. It can also have a negative impact on the children who witness the abuse.
Question 5: How can I get help if I am being abused?
If you are being abused, it is important to get help. There are many resources available to help you, including:
- Local domestic violence shelters
- National domestic violence hotline: 1-800-799-SAFE
- Online resources, such as the National Coalition Against Domestic Violence website
Question 6: How can I help someone who is being abused?
If you know someone who is being abused, there are things you can do to help:
- Encourage them to get help
- Provide them with information about local resources
- Offer them emotional support
- Help them to develop a safety plan
Elvis abuse is a serious issue, but it is one that can be overcome. With the help of caring friends, family members, and professionals, victims of Elvis abuse can heal from their trauma and rebuild their lives.
If you or someone you know is experiencing Elvis abuse, please reach out for help. There are many resources available to help you, and you are not alone.
Tips for Addressing Elvis Abuse
Elvis abuse is a serious issue that can have a devastating impact on the lives of those who experience it. It is important to be aware of the signs and symptoms of Elvis abuse, and to know how to get help if you or someone you know is being abused.
Here are five tips for addressing Elvis abuse:
Tip 1: Educate yourself about Elvis abuse.The first step to addressing Elvis abuse is to educate yourself about the issue. Learn about the signs and symptoms of abuse, the causes and consequences, and the resources available to help victims.Tip 2: Believe survivors.
If someone tells you that they are being abused, believe them. Do not dismiss their experiences or try to minimize the abuse.Tip 3: Offer support.
Victims of Elvis abuse need support from their friends, family, and community. Offer them emotional support, practical assistance, and information about resources.Tip 4: Encourage them to get help.
If someone is being abused, encourage them to get help. There are many resources available to help victims of abuse, including domestic violence shelters, hotlines, and online resources.Tip 5: Hold abusers accountable.
Abusers must be held accountable for their actions. If you know someone who is abusing someone else, report it to the authorities.
Elvis abuse is a serious issue, but it is one that can be overcome. With the help of caring friends, family members, and professionals, victims of Elvis abuse can heal from their trauma and rebuild their lives.
If you or someone you know is experiencing Elvis abuse, please reach out for help. There are many resources available to help you, and you are not alone.
Conclusion
Elvis abuse is a serious issue that can have a devastating impact on the lives of those who experience it. It is important to be aware of the signs and symptoms of Elvis abuse, and to know how to get help if you or someone you know is being abused.
This article has explored the various aspects of Elvis abuse, including its definition, causes, consequences, and resources for getting help. It is important to remember that Elvis abuse is never the victim's fault, and that there is help available for those who are experiencing it. If you or someone you know is being abused, please reach out for help. There are many resources available to help you, and you are not alone.
Unveiling The Truth: The Rise And Fall Of Alex Rodriguez And Cynthia Scurtis' Marriage
Unveiling The Secrets Of Daryl Sabara's Relationships: A Journey Of Love And Acceptance
Unlock The Secrets Of Robby Benson's Enduring Family Legacy
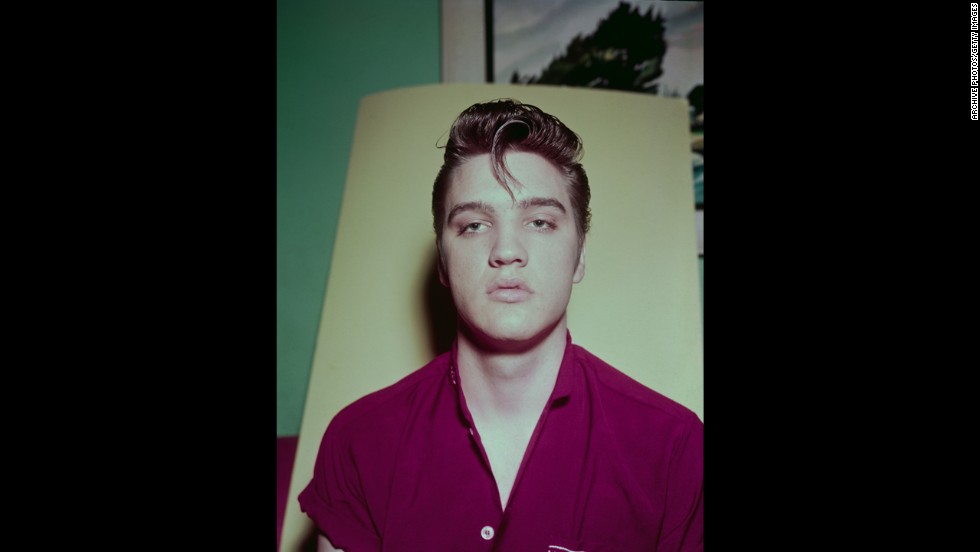
Bob Crane murder We still don't know who did it CNN
Why Did Elvis & Priscilla Presley Divorce? The Real Reason They Split
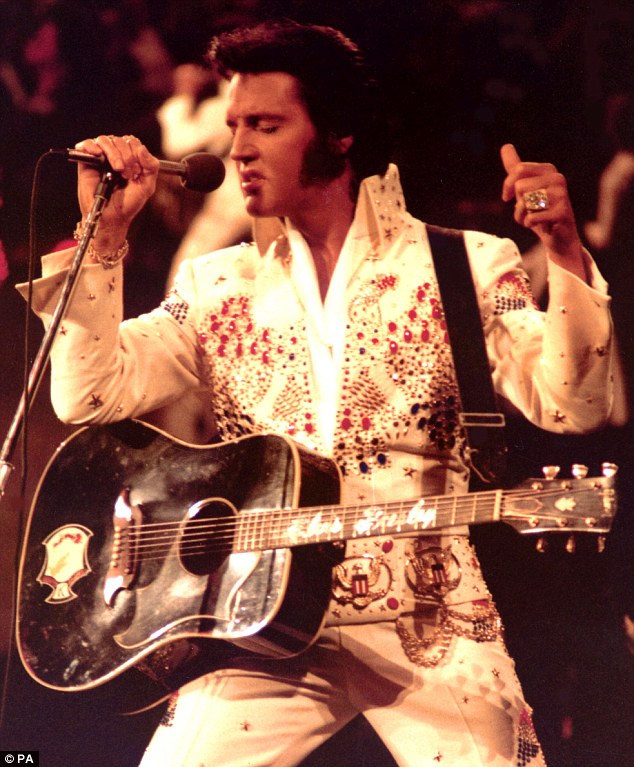
Pill bottles that held Elvis's painkillers go up for auction in New
ncG1vNJzZmiamaO8r7ONmqSsa16Ztqi105qjqJuVlru0vMCcnKxmk6S6cLHLr6CsZZGXwrSxjaGrpqQ%3D